Let’s say you are writing a shell script for a systems housekeeping task. Perhaps the script is uploading backups to a remote server, or it is cleaning up old data, or it is making a measurement and submitting it to a somebody else’s HTTP API. What is the least cumbersome way for the script to contact you in case of problems? Let’s say you want to be contacted via email – how to make it work?
Cron and MAILTO
If you run the script from cron, you can look into using cron’s MAILTO= option. You put a MAILTO=you@example.org
line in your crontab, and, when a job fails, cron will send a notification to the specified address using system’s MTA. OK, then, what MTA to use?
sSMTP
sSMTP is a send-only MTA which hands off messages to an external SMTP server that you configure in /etc/ssmtp/ssmtp.conf
. For example, you can create a dedicated Gmail address for sending notifications, and use it in ssmtp.conf
like so:
Root=username@gmail.com
Mailhub=smtp.gmail.com:465
AuthUser=username@gmail.com
AuthPass=google-app-password-here
UseTLS=YES
Gmail-specific note: for the Gmail SMTP service to accept your credentials, you will need to set up and use an app password. To use app passwords in your Google account, you will also need to set up 2-step-verification. The app password must be guarded with the same care as your account’s main password, so putting it in ssmtp.conf
is not ideal. This is why I would strongly recommend to use a separate Gmail account, not your main account, for this.
With sSMTP installed and configured, sSMTP will pass messages on to Gmail, and Gmail will deliver them to your inbox. This will make cron’s MAILTO option just work, and you can then also send messages from shell scripts using the mailx
program:
echo "Hello world" | mailx -s "Subject goes here" you@example.org
Similar sSMTP alternatives are nullmailer and msmtp.
I used Gmail as example, but if you use a different email provider, it likely provides an SMTP interface as well. Transactional email services also typically provide an SMTP interface. For example, I’ve used sSMTP with Mailgun, and it works great. In short, sSMTP needs working SMTP credentials – it does not matter if they are from Fastmail, Zoho, AWS SES, Sendgrid, Mailjet or something else.
HTTP API
The transacional email services usually provide a HTTP API. Depending on the provider, the API can be so simple you can use it with a simple curl call. Here’s an example with the already mentioned Mailgun:
curl --user "api:your-api-key-here" \
https://api.mailgun.net/v3/yourdomain.com/messages \
-F from='sender@yourdomain.com' \
-F to='you@example.org' \
-F subject='Subject goes here' \
-F text='Message body goes here'
The upside of this approach is you don’t need to install and configure anything on your server beforehand (assuming curl is preinstalled). One downside is you cannot use this method with cron’s MAILTO, as curl is not a MTA. But you can use this method just fine from scripts.
HTTP API is also easy to use from a Python script:
import os
import requests
def email(subject, text):
url = "https://api.mailgun.net/v3/yourdomain.com/messages"
auth = ("api", os.getenv("MAILGUN_KEY"))
data = {
"from": "sender@yourdomain.com",
"to": "you@example.org",
"subject": subject,
"text": text,
}
requests.post(url, auth=auth, data=data)
Hosted Log Management Systems
Here, I’m thinking of systems like LogDNA and Papertrail. You configure your system’s syslog to ship system logs to your chosen log management system. In that system, you set up alerting rules like “alert me if this specific keyword appears in logs this many times in this long time window”. And that is all!
Logging to syslog from scripts is easy using the logger
command:
logger Hello World!
As an example, here’s a notification I received from Papertrail a few days ago. It runs a saved search once per hour and sends a notification if the search produces any results:
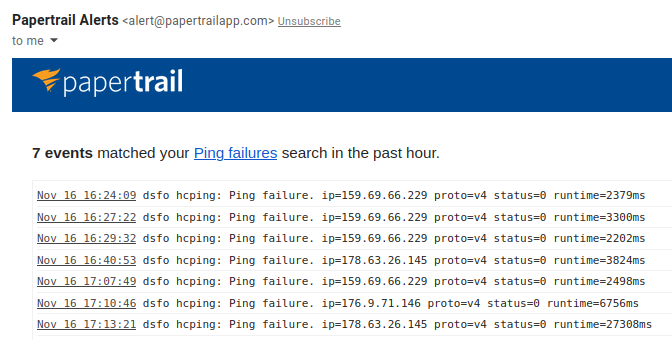
Cron Monitoring Services
And here I am of course thinking of Healthchecks.io, but there are good alternatives too. The monitoring service provides an unique URL that the housekeeping script must request regularly. When an HTTP request from the script does not arrive on time, Healthchecks.io detects that and alerts you. This takes care of scenarios, where, for example, the server has been shut down, and so is unable to contact you by itself. Healthchecks.io offers a lot more features, but this is the basic idea.
Here’s an example notification I received from Healthchecks.io. My home router is pinging Healthchecks.io. When home connection goes down, I get an alert:
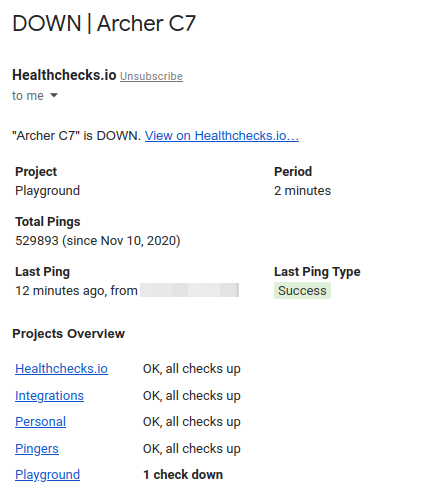
In summary:
- sSMTP is a good choice for use in combination with cron’s MAILTO option
- HTTP APIs are handy for sending emails from scripts, without needing to install or configure any additional software
- Log management systems and cron job monitoring systems can send email notifications, but they specialize in specific tasks. Log management systems can notify you about patterns in log files, cron job monitoring systems can detect irregularities in the heartbeat signals sent by your background tasks.